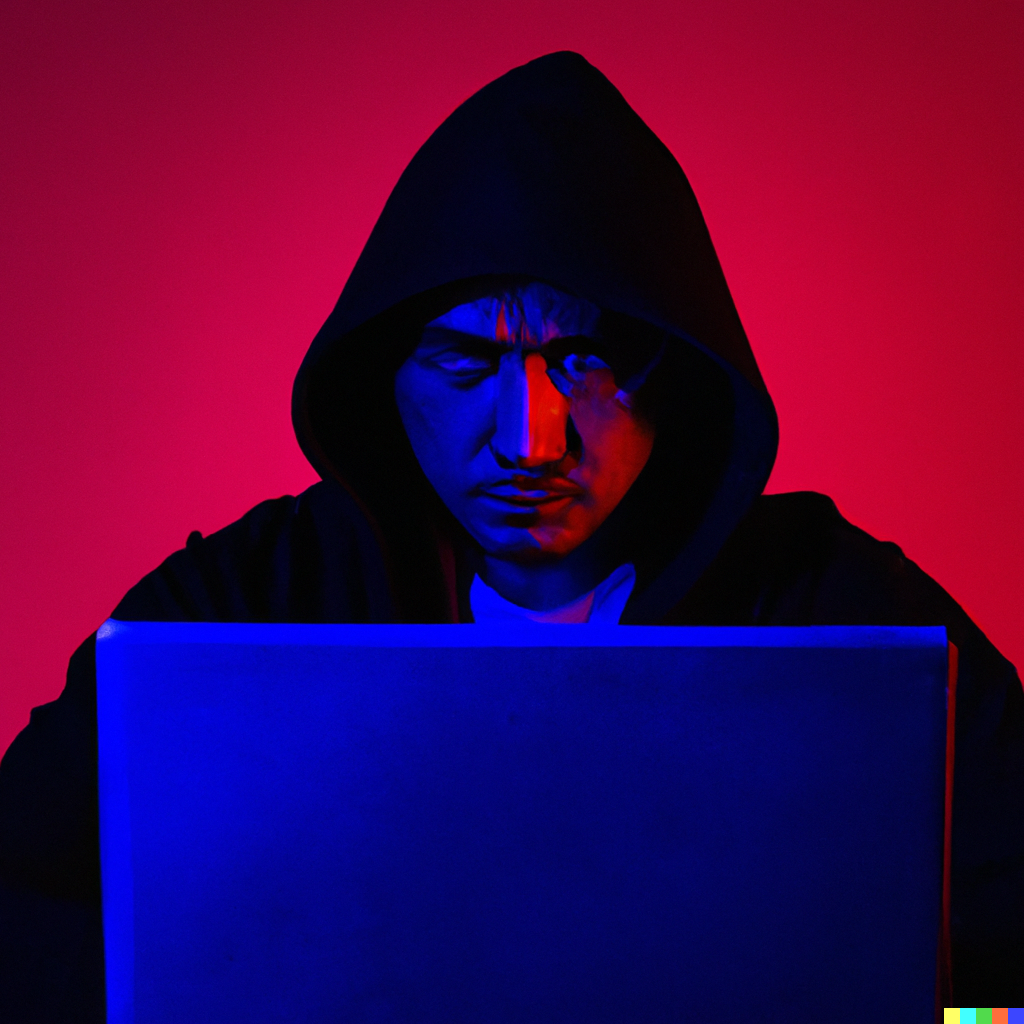
The Win32_Service Class and VBScript
For background, the Win32_Service
class is part of the Windows Management Instrumentation (WMI) system, and it allows you to interact with Windows services programmatically. Here’s an explanation of how to use it in VBScript:
' Create a WMI service object
Set objWMIService = GetObject("winmgmts:{impersonationLevel=impersonate}!\\.\root\cimv2")
' Query for a specific service (e.g., "Spooler")
Set colServices = objWMIService.ExecQuery("SELECT * FROM Win32_Service WHERE Name='Spooler'")
' Iterate through the collection of services (should be just one in this case)
For Each objService In colServices
WScript.Echo "Service Name: " & objService.Name
WScript.Echo "Display Name: " & objService.DisplayName
WScript.Echo "Status: " & objService.Status
Next
In this example, we first create a WMI service object using GetObject
with the appropriate namespace (root\cimv2
). Then, we use ExecQuery
to run a WQL (WMI Query Language) query to select a specific service by its name. In this case, we are querying the “Spooler” service. You can replace “Spooler” with the name of the service you want to query.
Finally, we iterate through the collection of services (which should have only one service in this example) and display some properties of the service, such as its name, display name, and status.
This is just a basic example, and you can perform various actions with Windows services using Win32_Service
through WMI, such as starting, stopping, or modifying services, depending on your needs.
Opening Apps Remotely with VBScript
If you’re ever find yourself in need of opening a Windows application remotely on a network computer you can try this handy VBScript.
In the example below, run this script “as is” to see that it will open Notepad.exe on your local computer.
Next, to get this to open notepad.exe on a remote computer on the same network, change the strComputer variable from “.” to the name of your network c0mputer.
In the example below, I’ve copied a text file to to the remote computer, C:\scripts\test.txt, and we’ll open it with notepad on the network computer so the remote user can read our message.
Sample Code: Open NotePad Remotely
'Pass a . to run this on your own PC or a string value for a network computer
strComputer = "."
Set objWMIService = GetObject("winmgmts:" _
& "{impersonationLevel=impersonate}!\\" & strComputer & "\root\cimv2:Win32_Process")
Error = objWMIService.Create("notepad.exe 'C:\scripts\test.txt'", null, null, intProcessID)
If Error = 0 Then
Wscript.Echo "Notepad was started with a process ID of " _
& intProcessID & "."
Else
Wscript.Echo "Notepad could not be started due to error " & _
Error & "."
End If
Troubleshooting
If you’re having problems getting this script to run on a corporate network, you may need to run this script as an admin. If you don’t know how to do that, check out my article on how to run VBScript as an adminstrator.
I’ve recently run into a situation where I needed this script to send a message to users on remote Kiosk PCs that don’t require authentication in another city on a large corporate network. This script would do the trick.
I hope this article helped you!
~Cyber Abyss