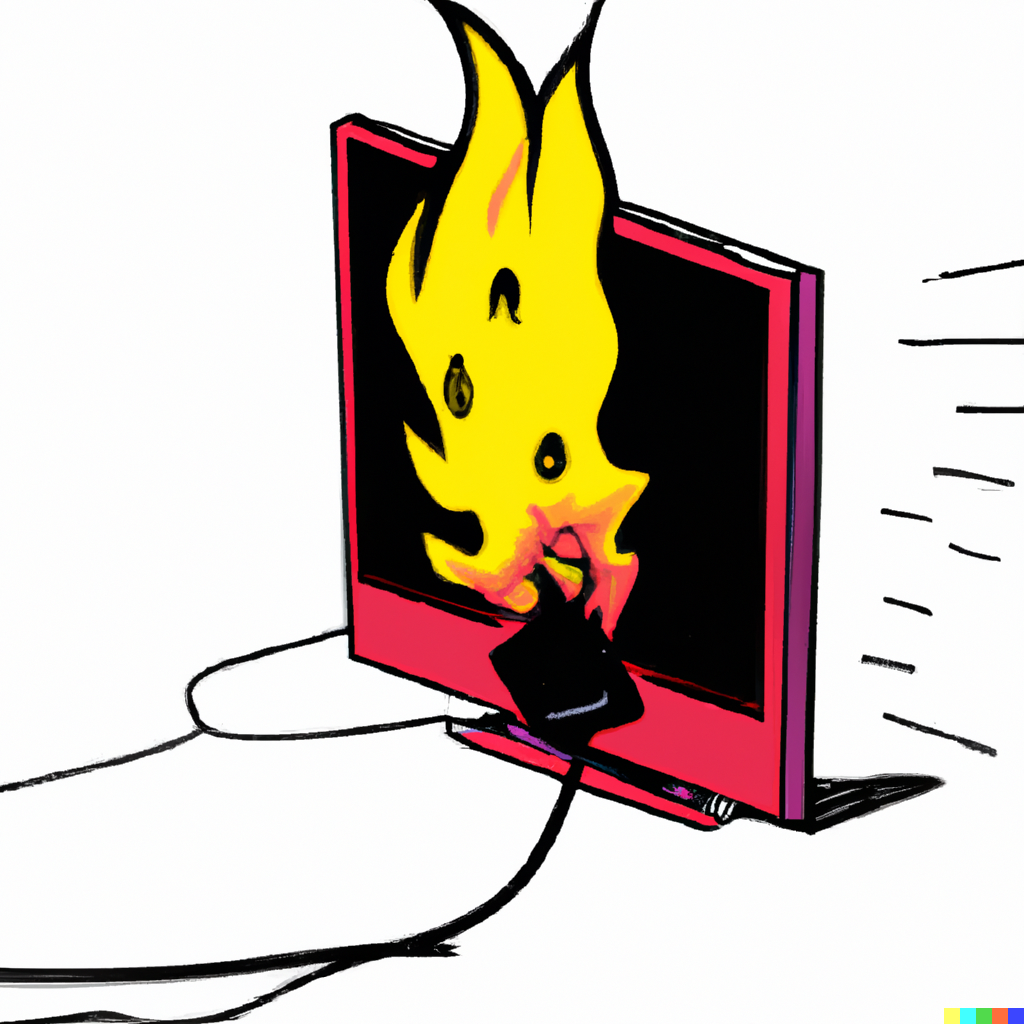
I’m taking a class on how to build Arduino devices.
This morning’s Arduino lesson used a temperature sensor to read the temperature and output it to the Arduino serial monitor.
Here is my Arduino code which is a combo of code from the class plus my attempt to integrate an alarm to it which I got from a combination of YouTube and Arduino online resources.
The code is my own creation based on stuff I found.
It is free for you to use copy improve and share. Enjoy!
Arduino Based Temperature Monitor Sample Code
int tempPin = A1; //Sets Analog Pin for Temp Sensor
int buzzer = 12; //Set alarm pin for peiezio sensor
int alertTemp = 78;
void setup() {
// put your setup code here, to run once:
Serial.begin(9600); //Starts serial monitor output
pinMode(buzzer,OUTPUT); //initialize the buzzer pin as an output
}
void loop() {
// put your main code here, to run repeatedly:
int sensorVal = analogRead(tempPin); //Reads the temperature sensor
float voltage = sensorVal * 5; //Turns sensrVal to Voltage
voltage /= 1024; //Divides voltage by 1024 and sets voltage to that result
float tempC = (voltage - .5) * 100; //Determines temp inCelsius from Voltage
float tempF = (tempC * 9 / 5) + 32; //Determines temp in Fahrenheit from Celsius
/***************** Begin Loop for alarm if temp is above ***********************/
unsigned char i; //define a variable
if(tempF > alertTemp) {
Serial.println(tempF); //Prints value of tempF variable anbd end line
Serial.print("Celsius Temp: "); //Prints Title
Serial.print(tempC);
Serial.print(" Fahrenheit Temp: "); //Prints Title
delay(1000); //delay for 1000 milliseconds
//output an frequency
for(i=0;i<80;i++) {
digitalWrite(buzzer,HIGH);
delay(1);//wait for 1ms
digitalWrite(buzzer,LOW);
delay(1);//wait for 1ms;
}
//output another frequency
for(i=0;i<100;i++){
digitalWrite(buzzer,HIGH);
delay(2);//wait for 2ms
digitalWrite(buzzer,LOW);
delay(2);//wait for 2ms;
}
}
else {
digitalWrite(buzzer,LOW);
}
/***************** End Loop for alarm if temp is above ***********************/
Serial.println(tempF); //Prints value of tempF variable and end line
Serial.print("Celsius Temp: "); //Prints Title
Serial.print(tempC);
Serial.print(" Fahrenheit Temp: "); //Prints Title
delay(1000); //delay for 1000 milliseconds
}