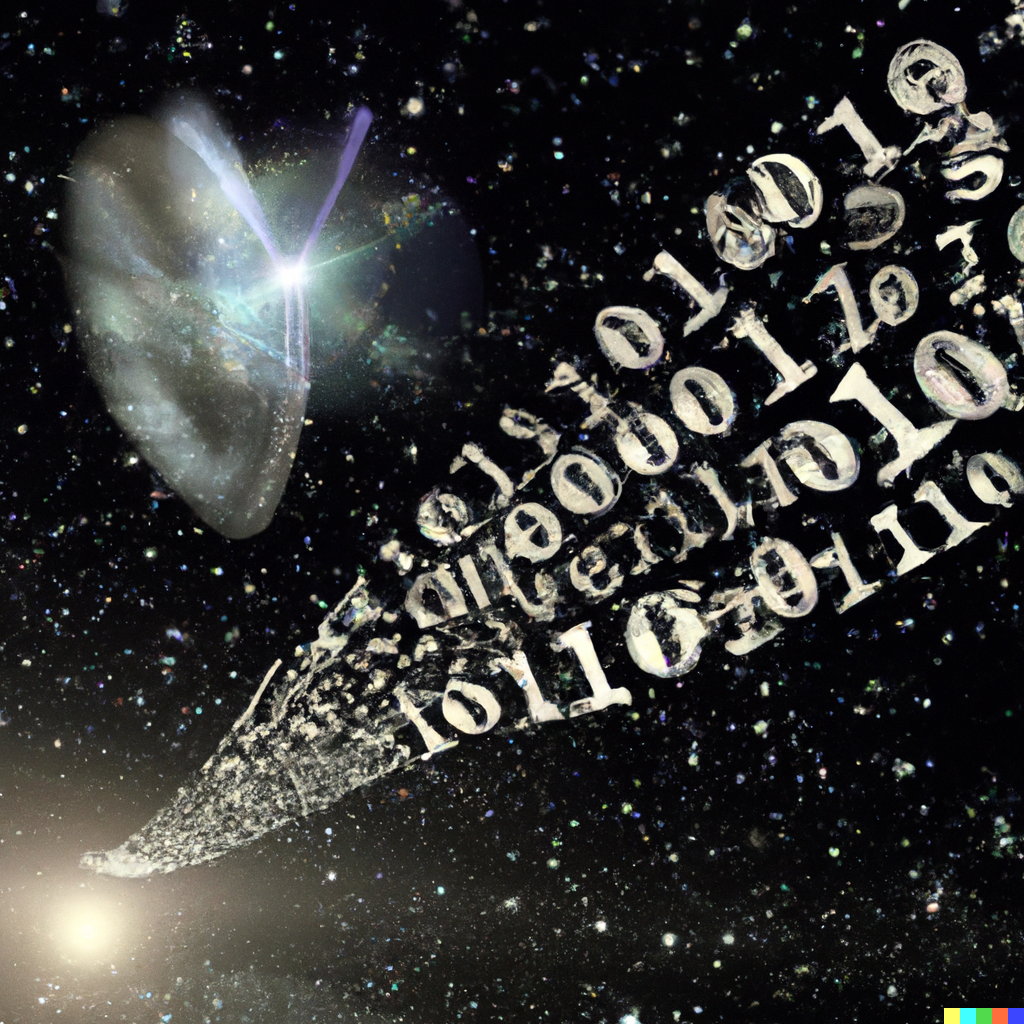
Database Table Backups on the Fly
This is a super simple SQL super power trick I learned from a mentor.
If you’re working in SQL Server Management Studio (SSMS) you can open up a New Query window and type in a simple “SELECT INTO” command to backup a database table on the fly.
Sample SQL SELECT INTO Statement
SELECT * INTO new_table FROM old_table_20221122
This is pretty simple. You’re making copying of a table like a “Save As” button would let you do.
This is also great for undoing something bad you’ve done by simply reversing the process.