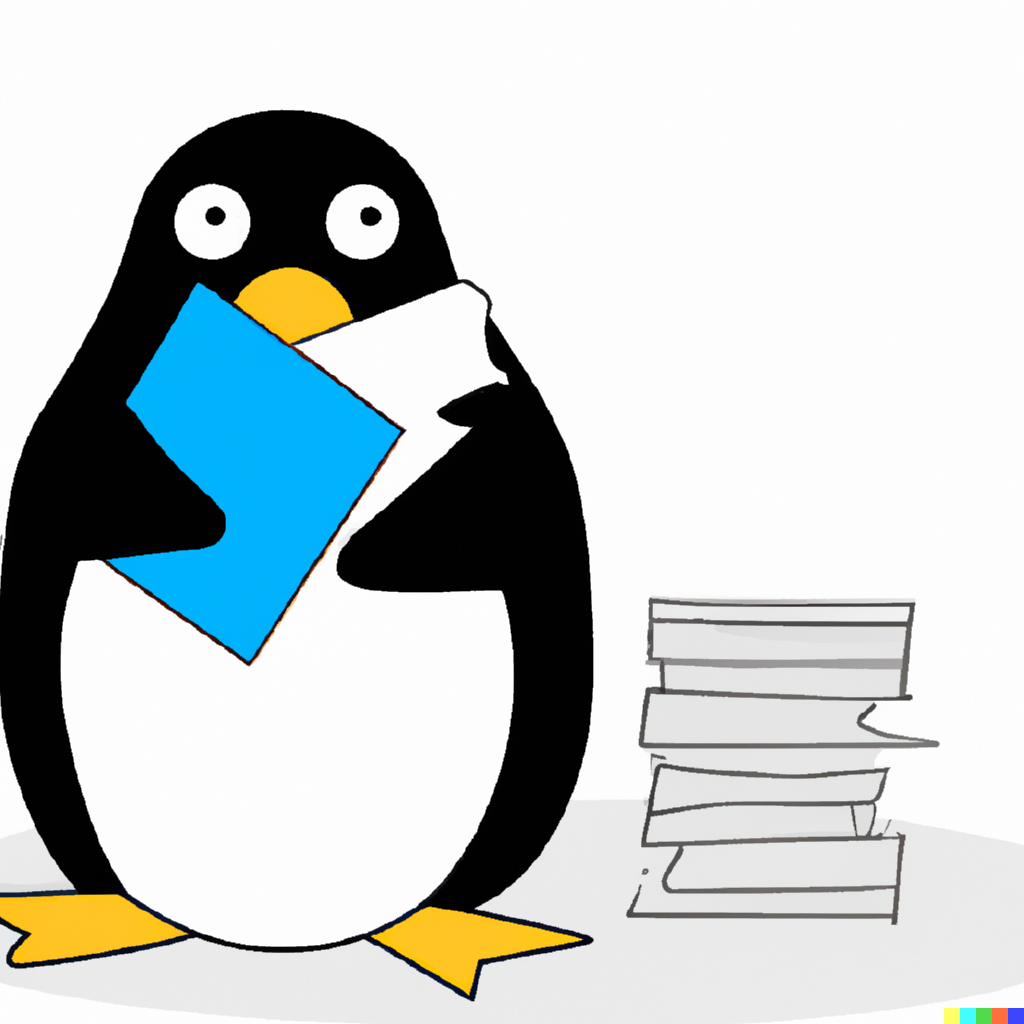
I’m want to look at my cloud hosted Linux Apache web server log files on my local Windows PC and I’m using PuTTY SSH as my Windows SSH telnet client.
How do we transfer the Apache web server log files over to my local Windows PC so I can work with it?
Instead of trying to copy the file from inside the PuTTY SSH session on the remote server, we can use a built-In Windows command line tool (pscp.exe) included with PuTTY called “PSCP” which mimic much of what you get with Linux’s built-in scp file transfer utility.
PuTTY
PuTTY is a free and open-source terminal emulator, serial console, and network file transfer application. It supports various network protocols, including SCP, SSH, Telnet, rlogin, and raw socket connection. It can connect to a remote server or computer over these protocols to securely execute commands at the terminal over the network, manage files, or tunnel other applications. PuTTY is most commonly used on Windows, but versions for Linux and macOS are also available.
PuTTY was originally written for Microsoft Windows but now has versions for other operating systems. It is widely used by network administrators and other users who need to remotely manage systems and devices or transfer files securely.
PSCP
PSCP stands for PuTTY Secure Copy Client, a command-line tool that allows you to securely transfer files between computers using the SCP protocol. PSCP is part of the PuTTY suite of tools and is used for non-interactive file transfers from the command line. It is compatible with both Windows and Unix-like operating systems.
With PSCP, you can upload files from your local machine to a remote server or download files from a remote server to your local machine using a secure connection. It’s particularly useful for automating file transfers in scripts or for environments where a graphical user interface is not available.
Here are some basic examples of how PSCP is used:
- To copy a file from your local machine to a remote server:rubyCopy code
pscp localfile.txt user@remotehost:/remote/directory
- To copy a file from a remote server to your local machine:rubyCopy code
pscp user@remotehost:/remote/file.txt localdirectory
In these commands, user
represents the username on the remote host, remotehost
is the address of the remote server, and localdirectory
is the directory on your local machine where you want to copy the files.
Remember, when using PSCP, you’ll be prompted for passwords or you can use SSH keys for authentication, depending on your server’s configuration and your setup.
My Personal Experience with Putty & PSCP
Putty’s Command Line File Transfer Tool (PSCP)
Below is a screenshot of me figuring out how to properly transfer an Apache web server log file over to my Windows PC temp folder on the C drive.

Sample Command Line Code
Run from the Windows Command Line from your Putty Install folder.
I used // to escape the forward slashes on the Linux server and \\ to escape backslahes and it worked.
Below should be entered all on one line.
c:\Program Files\PuTTY>pscp user@remoteserver://var//log//apache2//access.log c:\\temp\\access.log
Summary
You can use Putty’s PSCP command line tool to copy files from your Linux host to your Windows PC with these basic command line commands.
I hope this article helped you solve your problem!
~Cyber Abyss