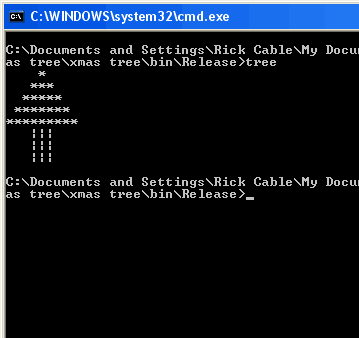
Below is the original article I wrote back in 2009 but find the topic still relevant for students new to C#.
Introduction to C# Programming: ASCII XMas Tree – C# and .Net Sample Project.
C#, pronounced C Sharp, is a relatively new programming language from Microsoft that was designed to take full advantage of the new .Net Framework.
Below is a sample project I did for a programming class I’m attending at MJC in Modesto. To test and run the code listed on this site you should download a free copy of Microsoft Visual C# Express 2005. There are four Visual Studio Express versions available for free from Microsoft, Visual Basic, C#, J# and C++.
The X-Mas tree project was a good idea because it really makes you think.
See the code example below.
C# ASCII XMas Tree Code Sample
using System;
using System.Collections.Generic;
using System.Text;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
//create the main array
int[] myArray = new int[] { 1, 3, 5, 7, 9 };
//The outside foreach loop to loop throught the array
foreach (int intLoop in myArray)
{
//creates the spaces, takes the array number minus 1 then divide by 2
//this gives you the amount of spaces needed for each level of the tree
for (int iSpace = 0; iSpace < ((myArray[4]-intLoop)/2); iSpace++)
{
System.Console.Write(" ");
}
//middle loop writes the asterisks "*" the full amount of current array[]
for (int i = 0;i < intLoop; i++)
{
System.Console.Write("*");
}
//creates the spaces, takes the array number minus 1 then divide by 2
//this gives you the amount of spaces needed for each level of the tree
for (int iSpace = 0; iSpace < ((myArray[4] - intLoop) / 2); iSpace++)
{
System.Console.Write(" ");
}
//creates new lines after all 3 loops run
System.Console.WriteLine("");
}
//nest this loop and do it 3 times
for (int iBase = 0; iBase < myArray[1]; iBase++)
{
// now make the base of the tree
for (int iSpaces = 0; iSpaces < myArray[1]; iSpaces++)
{
System.Console.Write(" ");
}
for (int iPipes = 0; iPipes < myArray[1]; iPipes++)
{
System.Console.Write("|");
}
// now make the base of the tree
for (int iSpaces = 0; iSpaces < myArray[1]; iSpaces++)
{
System.Console.Write(" ");
}
//creates new lines after all 3 loops run
System.Console.WriteLine("");
}
}
}
}
Good luck to you on all your future programming challenges!